Just add the pod "Squall" to your podfile, run pod install and you should be good to go.
Make sure your deployment target is at least iOS 8 or higher.
Example:
platform :ios, '8.0'
use_frameworks!
target 'MyApp' do
pod 'Squall'
end
You can also get all the latest changes straight from the Github repo by using:
pod 'Squall', :git=> 'https://github.com/marcuseckert/Squall'
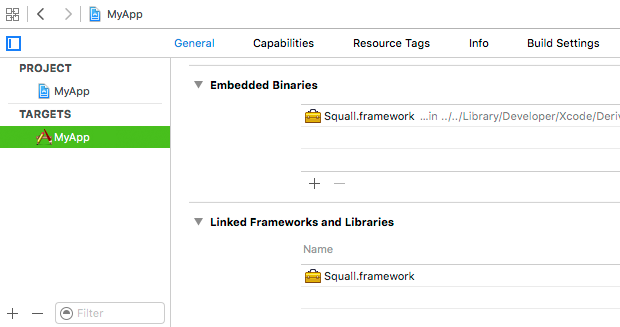
To fix this, add a "Run Script Phase" at the end of the build phases to your target and paste in the code from this link. This should strip all non-valid architectures from the frameworks in the framework folder of your build target before archiving the app.
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[Squall setLicenseKey:@"YOUR-LICENSE-KEY"];
return YES;
}